IB
Computer Science year 1 - Frankfurt
International School - Dave_Mulkey@fis.edu - 2015-16
IBIO
and Other Suggestions - 21 June 2016
If you want to use the IB standard input and output commands,
you can copy this code into your program:
https://dl.dropboxusercontent.com/u/275979/ibcomp/netbeans/IBIO.html
Make sure you put the code INSIDE the class you are writing,
but outside any other methods.
However, you should try to start using JTextField controls for input,
and JTextField or JTextArea for output, like this:
double total = 0;
private void
jButton1ActionPerformed(java.awt.event.ActionEvent
evt)
{
String inp =
jTextField1.getText();
if(inp.length()==0)
{
output("You need to type some data");
return;
}
double num =
Double.parseDouble(inp);
total = total + num;
jTextField2.setText(total + "");
String data =
jTextArea1.getText();
data = data + inp +
"\n";
jTextArea1.setText(data);
jTextField1.setText("");
jTextField1.requestFocus();
}
//===========================================================
//=== IBIO simplified input/output commands - GUI version
===
//===========================================================
public void output(String message)
{
javax.swing.JOptionPane.showMessageDialog(null,message); }
public void outputString(String message)
{ output(message); }
public void output(char info)
{ output(info + ""); }
public void output(byte info)
{ output(info + ""); }
public void output(int info)
{ output(info + ""); }
public void output(long info)
{ output(info + ""); }
public void output(double info)
{ output(info + ""); }
public void output(boolean info)
{ output(info + ""); }
//----- Numerical input methods return 0 on error
------------
public String input(String prompt)
{ return
javax.swing.JOptionPane.showInputDialog(null,prompt); }
public String inputString(String prompt)
{ return input(prompt); }
public String input()
{ return input(""); }
public char inputChar(String prompt)
{ char result=(char)0;
try{result=input(prompt).charAt(0);}
catch (Exception e){result = (char)0;}
return result;
}
public byte inputByte(String prompt)
{ byte result=0;
try{result=Byte.valueOf(input(prompt).trim()).byteValue();}
catch (Exception e){result = 0;}
return result;
}
public int inputInt(String prompt)
{ int result=0;
try{result=Integer.valueOf(
input(prompt).trim()).intValue();}
catch (Exception e){result = 0;}
return result;
}
public long inputLong(String prompt)
{ long result=0;
try{result=Long.valueOf(input(prompt).trim()).longValue();}
catch (Exception e){result = 0;}
return result;
}
public double inputDouble(String prompt)
{ double result=0;
try{result=Double.valueOf(
input(prompt).trim()).doubleValue();}
catch (Exception e){result = 0;}
return result;
}
public boolean inputBoolean(String prompt)
{ boolean result=false;
try{result=Boolean.valueOf(
input(prompt).trim()).booleanValue();}
catch (Exception e){result =
false;}
return result;
}
//============================================================
//=========== end IBIO
=======================================
//============================================================
|
Functional
Prototype - 14-21 June 2016

Here is a video version of a similar idea: MacTini
--------
Okay, that was the Visual Prototype.
Now we need to work on a Functional Prototype -
that is a very simple, limited version of your Java program.
It should include a simplified (incomplete) version of
your intended User Interface, with a small set of
actually functional features. Best if it supports
some of your User Stories, but not absolutely required.
Looking ahead, here are all the IA Project Deadlines:
Aug 24, 2016 - Functional Prototype
Starting
Simple
Sep
16, 2016 - Stage A : Planning / Analysis
Collect User Stories, Write Goals
Software
Design Principles
Oct 28, 2016 - Stage B : Detailed Modular Design
Iterative
Techniques and Decomposition
Design
Outline DesignOverviewBlank.doc
Nov
30, 2016 - Working Program (Beta Version)
Guidance Notes (from old syllabus -
ignore details)
Jan 11, 2017 - Stage C : Development
Look at examples
Example
8 Computer Science for Kids in Java
Example
4 Mancala Game in Scratch
Example
3 4-Color Map in Python
**
Feb 8, 2017 - Finished IA Final Deadline (sections A-E) **
Assessment
Criteria (IBO)
Checklist
(IBO)
Feb 13 - Mar 11, 2017 : Personal Interviews with teacher, 20 min each
Review
Final Exam - 13 June 2016
Here
is a copy of the final exam, including correct answers.
Your next deadline for the IA Project is
This requires you to write a simple Java program,
implementing a UI and basic functionality for your project.
You may work on the functional prototype during class
this week and next week.
Study
- 30 May 2016
Study for your final exam.
Section
1.2 Quiz - 27 May 2016
Do your Quiz.
https://dl.dropboxusercontent.com/u/275979/ibcomp/notes/Section1-2quiz.html
Then you can start reviewing for the final exam:
- read this entire blog to remind yourself of all the things we
studies
- read
this sample final exam (from last year)
Ethical
Issues in Usability - 25 May 2016
Read these notes to prepare for your quiz:
syllabus
1.2.4 - 1.2.16
Here is a short but good video about UI :
https://www.youtube.com/watch?v=iViiyjaaiyE
When things go wrong, is it only annoying,
or can there be more drastic consequences?
Here is an interesting, current story:
http://www.bbc.com/news/technology-36367221
Usability
- 24 May 2016
We will discuss syllabus
1.2.12 - 1.2.16 about Usability and Human-Computer-Interface (HCI).
We will have a 30 min QUIZ on Fri 27 May
about Netbeans and Syllabus topic 1.2.4-1.2.16 .
** Don't forget that your Visual Prototype is due tomorrow, 25 May. **
Today's Notes
HCI = Human Computer Interface
Paradigms = Patterns = Concepts
- WIMP = Windows Icons Menues
Pointer
- Touch/Swipe/Pinch
- Buttons
Usability
- Consistency
- Habits - what you are used to
- Depends on people as well as
INDUSTRY STANDARDS
- Hardware
- Flexibility |
Images
in NetBeans - 20 May 2016
Here are some notes about putting images into a NetBeans program.
https://dl.dropboxusercontent.com/u/275979/ibcomp/netbeans/NetBeansImages.html
Continue
Cashier Project - 19 May 2016
Keep practicing with NetBeans -
make the program described on the paper hand-out.
We will have a 30 min QUIZ on Fri 27 May
about Netbeans and Syllabus topic 1.2.4-1.2.16 .
Intro
to NetBeans - 18 May 2016
Why
use NetBeans?
First
Time NetBeans
== Quick Summary for Beginners ==
- New Project
[File][New Project]
Java JavaApplication [Next]
Type a Project Name, unclick [create main class], [Finish]
- New Interface
Right-click on Project name (left)
New ... JframeForm
Give it a good name, [Finish]
- GUI Builder
Drag SWING controls onto your jFrameForm
- Properties
Use PROPERTIES (maybe right-click) to make it pretty
- Automation
Double-click on a Button
type Java commands for automation
- Run
Click the PLAY button to run the program
- Emergency Stop
If it gets stuck, stop with [Run][Stop Build/run]
Fast
Food Cash Register
[HL]
Trees - 17 May 2016
Discuss
TREES as illustrated in these notes : Trees
Introduction
We
might make it to this
sample program.
System Design Basics 1.2.7.... - 12/13 May 2016
Continue Design Basics from 1.2.7
Homework -
Download and install NetBeans IDE (Java SE) from:
https://netbeans.org/downloads/
We will use this a little bit next week, and you will start using it
for your project in June.
System Design Basics 1.2 - 11 May 2016
IBO
COURSE GUIDE (1.2)
WIKI
BOOKS 1.2
Software
Design Notes
Continue Visual Prototype - 9 May 2016
Continue working on your Visual Prototype.
Use the opportunity to ask questions and get help.
Don't forget to do your Homework Reading:
== HOMEWORK (to be done before Wednesday) ==
Read this : https://dl.dropboxusercontent.com/u/275979/ibcomp/projects2014/softwareDesign.html
Take notes as you go, write down questions if you have them.
Starting the Visual Prototype - 4-9 May 2016
START drawing a VISUAL PROTOTYPE - this consists of INTERFACE SCREENS
that you can show your CLIENT when you discuss the project with them.
This might be jumping ahead a bit, but it's probably easier to have
something to show your client so they can say "Yes, I like that" or "No, it
needs to be different here."
When discussing this with your user, be sure to TAKE NOTES. You might
want
to PRINT your VISUAL PROTOTYPE so you can write notes on it during the
interview.
Here is another example Problem and Visual Prototype
The intention of this project is to REPLACE the
paper sheets
used for keeping track of students who leave
class. The paper
sheets are convenient for the signing in and out,
but they present
a large problem for the office. There are
100 teachers each turning
in 5 sheets each week to the office. Then
the secretary must read
all 500 sheets to collect information about
individual students.
An automated program should be able to sort out
the information
and present a clear list to the administration.
Visual Prototype - click here to see all the
pages: mockup.pdf
|
You can create your VISUAL PROTOTYPE right inside your PROJECT DOCUMENT
if you wish. Otherwise, you might wish to use PowerPoint to make the
VISUAL PROTOTYPE.
Here is a very nice TIC-TAC-TOE
prototype made slightly interactive in Powerpoint.
Yours probably does NOT need to be this good. |
But DO IT QUICKLY. It does not need to be "complete" or "perfect",
and does not need to be "interactive",
but it's good if it "tells a story" (or storIES). So like one click
to advance from one screen to the next is nice.
Your VISUAL PROTOTYPE is due on 25 May (and will be graded).
We will work on it TODAY and MONDAY during class, but after that
you must work outside class. Use this opportunity to ASK QUESTIONS
and GET HELP so you get off to a good start. If you want to talk to
your CLIENT
this month, please do.
== HOMEWORK (to be done before next Wednesday) ==
Read this : https://dl.dropboxusercontent.com/u/275979/ibcomp/projects2014/softwareDesign.html
Take notes as you go, write down questions if you have them.
More IA Projects - 3 May 2016
We will look at some sample projects in a bit more detail,
as well as discussing Creeping Featurism and Criteria
for Success (GOALS).
[HL] More Lists - 2 May 2016
You might want to read about STACKS in Data
Structures .
You might also like to read about Reverse Polish Notation:
http://www.hpmuseum.org/rpn.htm
import java.awt.*;
import java.util.*;
public class Stacks extends GUI
{
LinkedList<String> stack = new
LinkedList<String>();
Button start =
addButton("Start",50,50,100,50,this);
public static void main(String[] args)
{
new Stacks();
}
public Stacks()
{
super(400,300);
}
public void actions(Object source, String
command)
{
String item = "";
if(source == start)
{
stack.removeAll(stack);
do
{
item = input("Input");
if(item.equals(""))
{
output("Result = " + stack.getLast());
}
else if(item.equals("+"))
{
double a = Double.parseDouble( stack.getLast() );
stack.removeLast();
double b = Double.parseDouble( stack.getLast() );
stack.removeLast();
double value = a+b;
stack.addLast( value + "" );
}
else if(item.equals("*"))
{
double a = Double.parseDouble( stack.getLast() );
stack.removeLast();
double b = Double.parseDouble( stack.getLast() );
stack.removeLast();
double value = a*b;
stack.addLast( value + "" );
}
else
{
stack.addLast(item);
}
}while(item.length()>0);
}
}
} |
You need to create this class in a BlueJ project
that already contains the GUI class.
User Stories = Scenarios = Use-Cases - 29 Apr 2016
When you start discussing your PROBLEM with your CLIENT, remember to TAKE
NOTES!
Try to get the CLIENT to tell you stories. User
Stories often start with WHEN:
- When I go shopping, I try to find the items that are on sale.
Then I might buy several packages.
- When a student leaves school early, they need to type
their name, the time, and the reason for leaving.
- When the Tic-Tac-Toe board is full, then the game is over
and it is a tie (no winner).
Here is what Martin Fowler says about user-stories (in Extreme
Programming):
http://www.martinfowler.com/bliki/ConversationalStories.html
More User Stores in the following documents:
Software
Design Principles (section: Current System Stories)
Starting
Simple (look at: Visual Prototypes)
Complete
Overview (p. 6 - 7)
You are going to do lots of things while building this project.
You really NEED to:
- work on various parts of the project in parallel
- keep WRITTEN NOTES of what you are thinking and doing
So as you are sitting and thinking about your PROBLEM and CLIENT and
AUTOMATION,
you are likely to have some IDEAS of how you will be solving the problem.
Write these down as notes - keep the notes in a document.
If you prefer paper, that's fine, but KEEP the notes.
Right now, try to think in terms of stories - but even rough notes will be
helpful.
IA Project Overview : Importance of Intended User - 28
Apr 2016
Look at the first section in Software
Design Principles
and at PAGE 4 in Guidance Notes (from old syllabus -
ignore details)
Choosing a Problem and Client (intended user) - 27 Apr
2016
You need to choose a PROBLEM and a CLIENT.
You need both - they fit together.
You must write a ONE PARAGRAPH explanation of
your problem and client and turn this in on Monday 2 May.
The explanation must include a SUGGESTION of how
AUTOMATION in the program will IMPROVE something
for the CLIENT (intended user).
Today - read these suggestions :
Starting
the Project - Suggested Topics (ignore the dates)
Very Good Example 1: (grade 7)
My client will be Mr Mammoth, a math teacher at Fantasy Island
School.
The problem is that Mr Mammoth's students forget to do their
homework.
The solution will create online math problems for the students to
do,
which will make homework more attractive, interesting and effective.
The automation will be:
1 - automatically generate a variety of problems based on a
template,
but with different numbers each time.
2 - every problem will be accompanied by a picture,
3 - the program will mark the student's solution right/wrong.
~~~~~~~~~~~~~~~
-- Excellent! --
|
Good Example 2 : (grade 6)
My client will be the basketball coach, Ms Bouncy.
She needs to keep track of personal information about her players,
especially the number of points they score in each game.
It needs to add up all the points for the entire season for each
player.
~~~~~~~~~~~~~~~
-- It's a good start, but is too simple as it is stated --
-- That's alright, as long as the programmer will
--
-- be able to think of more automation, because --
-- just adding up player's scores is not
enough. --
|
Okay Example 3 : (grade 4)
My client will be my mother.
My program will keep track of all the money she spends shopping
for food. The automation will be to search for items in a
specific
category and add up the total cost.
~~~~~~~~~~~~~
-- need the client's
name
--
-- there is no statement of the PROBLEM --
-- the automation described is too simple --
|
Bad Example 4 : (grade 3)
I am going to make a program that all students will like.
It will keep track of their schedule. It will be user
friendly.
~~~~~~~~~~~~~
-- You must have ONE SPECIFIC
CLIENT
--
-- As described, there is no PROBLEM
because
--
-- every student already has a copy of their
--
-- schedule, on paper, and this is very efficient.
--
-- So we need to know what AUTOMATION is intended --
-- that will improve the
situation.
--
|
Starting IA Project - 25 Apr 2016
We will have the last student group presentation,
then briefly discuss how videos can be better designed.
Then we will discuss the Internal
Assessment Project Notes.
Group 4 Project - Algorithms - 11 Apr - 22 Apr 2016
Read
the assignment here.
Stacks and Queues in Pseudocode - 24 Mar 2016
Use the Pseudocode
practice tool to investigate
Stacks and Queues.
Homework
Look at the STACK program and the QUEUE program in Pseudocode.
For each, write an equivalent JAVA program.
You probably want to use a LinkedList in each case.
You might find this document helpful: Pseudocode2
Pseudocode Practice - 23 Mar 2016
To practice and learn Pseudocode, you should practice READING it.
The best way is as follows:
- open a sample program in the Pseudocode
practice tool
- run the program and TEST it with various data
- once you understand what it does,
write the same program in Java (using BlueJ)
If you wish, you can COPY the Pseudocode program
and paste it into BlueJ, then might minor changes to make it
work in Java
- Repeat this for other sample programs
Be sure that you do at least one SORT program and one SEARCH
program
Here are 2 IBO Documents that might be helpful:
Pseudocode1
Pseudocode2
Algorithms - 22 Mar 2016
We will discuss topic
4.2 starting tomorrow.
You should plan on reading sections 7.1-7.5 in your
textbook before
returning to school after the break on 11 April 2016.
We will discuss PSEUDOCODE and use this Pseudocode
Tool.
Algorithms - 21 Mar 2016
Your TextWorker project is due TODAY at 23:59 CET.
You must:
- compress the FOLDER containing your program into a .ZIP archive
No, do NOT use a different archive format.
If you do not know how to do this, get help.
- upload the .ZIP archive into the Haiku Assignment Dropbox.
If you do not know how to do this, get help.
== Next Topic ==
We will discuss topic
4.2 starting tomorrow.
You should plan on reading sections 7.1-7.5 in your
textbook before
returning to school after the break on 11 April 2016.
TextWorker Project - 11-21 Mar 2016
We will start a project based on this simple program : TextWorker.zip
This illustrates what happens when Google sends a "spider" to crawl a
web-site.
The spider must take all the text, extract the single words, and then post
those words in its search INDEX. When a search request asks for a
specific word(s),
Google searches through the INDEX to find documents where that word occurred
often.
There are many other criteria for choosing which web-pages to present at the
top of the search, but word-frequency is one of them.
Another example of text analysis is the Readability Index of a document.
A document with lots of big words and long sentences is generally less
more difficult to read than a document with small words and short sentences.
You might wish to read about it here:
https://en.wikipedia.org/wiki/Flesch%E2%80%93Kincaid_readability_tests
Another example of text analysis is to create the INDEX found at the back
of a book. There are numerous issues here:
- don't include entries for small words that occur very often, like "of" or
"and"
- keep track of the POSITIONS of every occurrence of a word
- alphabetize the resulting list of words
Another example of text analysis is COMPARING two texts. You might
want to:
- find words that appear in both
- search for long phrases/sentences that are identical (plagiarism)
Another example is creating a SUMMARY of a long article.
One way to do this is:
- keep the first paragraph
- then keep the first and last sentences of each paragraph
- keep the last paragraph
You should improve the TextWorker program to make it a useful tool
for examining a large amount of text. Your program is due
Monday 21 Mar.
We will work on this during all classes next week, but you will also
need to work at home. You may discuss some ideas with other students,
but DO NOT COPY ANY JAVA CODE from other students.
There are some obvious low-level tasks which need methods written, for
example:
- alphabetize the list of words
- sort the words according to HOW OFTEN they occur
- count duplicate words
- remove duplicate words
- calculate average word length
- find the longest word in the text
- search for specific words in the text
- search for specific words given only the first few letters of the
word
- find entire sentences
You should start this assignment by writing some of these methods,
which can then be used to accomplish bigger tasks.
You may choose to use arrays or LinkedLists or both, however you wish.
Videos about Machine Intelligence - 10 Mar 2016
We will discuss yesterday's video.
- How can machines use LISTS to store data and learn?
- How much data does a "learning" computer need to absorb and store?
- How many words do you know?
- How can we write a Java program that "learns"?
- How could a computer "learn" a foreign language?
Then we will the first 8 minutes of watch
this video during class.
Then we will watch this entire video (maybe finish at home):
https://www.youtube.com/watch?v=mKZCa_ejbfg
We will discuss these videos on Friday during class.
Watch a Video - 9 Mar 2016
We are going to watch
this video (or at least part of it).
We will discuss this tomorrow during class.
Tomorrow we will watch
this video .
Then we will discuss that during class on Friday.
Quiz , Deleting from LinkedList - 7 Mar 2016
Start with a 5-10 minute quiz.
This program - InsertLists3.zip
- contains a pretty reliable INSERT algorithm.
It also contains a DELETE algorithm, which is not so reliable.
You need to fix the DELETE algorithm.
Quiz and More About Inserting - 4 Mar 2016
Start with a 5-10 minute quiz.
Then continue fixing InsertLists2.zip
Inserting in Lists - 3 Mar 2016
We will work on this program : InsertLists.zip
[HL] Queues - 2 Mar 2016
We will work on this sample program that illustrates
how QUEUES work - implemented in a LinkedList.
You might want to read about QUEUES in Data
Structures .
/******* Counselor Queues
**************
This program maintains two separate QUEUES
for teachers and students who are waiting
to see a Counselor.
The nextPerson button doesn't really work.
It just outputs "Go to lunch?"
Here is the PSEUDOCODE of how it should work:
- if there are any teachers waiting,
then output the name of the teacher
at the HEAD of the queue, then remove
this name from the teachers' Queue.
- If no more teaches are waiting,
then send in the next student -
if there are still students waiting.
- If both Queues are empty,
then send the Counselor to lunch.
This algorithm must work WITHOUT producing errors.
Also, there are some other problems that cause errors,
and these should be fixed.
*****************************/
import java.awt.*;
import java.util.*; // contains LinkedList class
public class Counselors extends GUI
{
public static void main(String[] args)
{ new Counselors(); }
Button student =
addButton("Student",50,50,100,50,this);
Button teacher =
addButton("Teacher",150,50,100,50,this);
Button nextPerson =
addButton("Next",250,50,100,50,this);
Button waiting =
addButton("Waiting",350,50,100,50,this);
LinkedList<String> studentsQ = new
LinkedList<String>();
LinkedList<String> teachersQ = new
LinkedList<String>();
public Counselors()
{
super(600,400);
}
public void actions(Object source, String
command)
{
if(source == student)
{
String who = input("Student name");
studentsQ.add(who);
}
if(source == teacher)
{
String who = input("Teacher name");
teachersQ.add(who);
}
if(source == nextPerson)
{
output("Go to lunch?");
}
if(source == waiting)
{
output(studentsQ.toString());
output(teachersQ.toString());
}
}
}
|
You need to create this class in a BlueJ project
that already contains the GUI class.
More About LinkedList - 1 Mar 2016
We will have a QUIZ (10-15 min) on
Friday 4 March
about LinkedLists, asking about the
commands
shown below. So you need to LEARN HOW THESE WORK
by performing experiments in BlueJ.
The QUIZ will be written on paper, without using a computer.
---------------------------------------------------------------
The following LinkedList commands
are required for the Paper 2 OOP exam.
Constructor: new
LinkedList<ElementType>()
.add(E e)
.add(int index, E element)
.addFirst(E e)
.addLast(E e)
.clear()
.element()
.get(int index)
.getFirst()
.getLast()
.remove()
.remove(int index)
.removeFirst()
.removeLast()
.size()
.isEmpty()
Here is the official documentation from Oracle (if that helps):
http://docs.oracle.com/javase/7/docs/api/java/util/LinkedList.html
JETS - Official Java Subset for IB Exams in OOP
Here are some general notes about Data
Structures .
LinkedList - 29 Feb 2016
The 4th version of Questions demonstrates a LinkedList:
Questions4.zip
A LinkedList is a COLLECTION that can store an arbitrarily large
number of Ojects, whereas an Array has a limited capacity.
Download the program, read the notes and do the Practice exercises.
Serialization - 19 Feb 2016
We will discuss SERIALIZATION as
demonstrated in this program:
Questions3.zip
Read the notes and do the practice exercises
at the end of the Questions class (program).
Discussing Questions Program(s) - 17 Feb 2016
Finish the Questions2 program that you started yesterday.
Especially interesting would be to change it to multiple-choice.
This would require 4 answers for each question.
Then these answers need to appear on 4 different buttons.
Finally, there must be another field in Question that tells
which of the answers is correct.
Questions OOP - 16 Feb 2016
Now we will look at a different version of Questions: Questions2.zip
This version uses a Question class, and an ARRAY of Question objects,
rather than using Parallel arrays.
Download Questions2.zip. Then read the notes and do the practice
exercises
at the end of the Questions class. It's probably best if you
work with another student.
Mr Mulkey will be back at work on Wednesday.
If you have any urgent questions before then,
you can send an email to : Dave_Mulkey@fis.edu
Javagain - 15 Feb 2016
We will start writing Java programs again
now with GUI and OOP and Arrays.
We will work on this program: Questions.zip
You should do the practice exercises
listed at the end of the Questions class (program).
Try to finish as much of this as possible today
(maybe work at home for 30 minutes)
because we will be working on a different
version of the program tomorrow.
[HL] Multi-tasking and Task Manager Experiments - 12 Feb
2016

== Today During Class ==
Discuss the questions on page
3 of this document.
Review Topic 2 - 10 Feb 2016
Here
are solutions for the Practice
exercises
***** WRITTEN TEST
TOMORROW (no computers) about Section
2 *****
Here are some review questions.
Here
are the ANSWERS to the review questions.
Other answers might be correct, but these are good answers.
More Circuits - 9 Feb 2016
Use the Circuit
Gates Emulator
to finish the problems on page 2 of Practice
exercises
.
Then write a Truth Table for this circuit WITHOUT using the emulator.
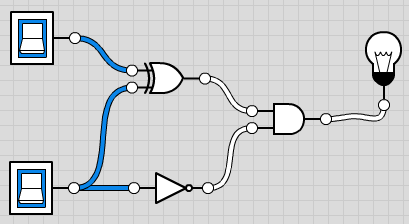
We will look briefly at : NAND
Gates and More Questions
Circuits - 5 Feb 2016
2.1.13
|
Construct
a logic diagram using AND, OR, NOT, NAND, NOR and XOR gates.
|
3
|
Problems
will be limited to an output dependent on no more than three
inputs.
The
gate should be written as a circle with the name of the gate
inside it. For example:

LINK
Thinking logically, connecting computational thinking and
program design, introduction to programming.
|
Not
enough space here
Logic
Gates Intro Video
Circuit
Gates Emulator
Practice
exercises
NAND
Gates and More Questions
|
Multi-tasking and Virtual Memory - 3-4 Feb 2016
Story : BOOT sequence
Story : 802.11x
We will discuss multi-tasking
and virtual memory,
then discuss questions from the last 2 classes.
== TEST next Thursday 11 Feb about Topic 2
==
Here
are brief notes about Topic 2 in the IB Syllabus
Here are some notes about hardware: Systems
and Hardware Pictures
[HL] More About Operating Systems - 2 Feb 2016
Homework from last time: Read
about Operating Systems

== Today During Class ==
Do the Practice Exercises on page
3 of this document.
IB Topic 2 - Microprocessors - 1 Feb 2016
Here
are brief notes about Topic 2 in the IB Syllabus
Here are some notes about hardware: Systems
and Hardware Pictures
== HOMEWORK ========================
Read some the notes above.
Write down or mark Vocabulary that is unfamiliar.
=================================
CPU Simulator - 29 Jan 2016
==
Homework for Thursday Evening and Friday Evening =================
Watch the FIRST HALF of each of the 4 videos below.
Sit with a pen and paper and WRITE DOWN questions
that arise during viewing. For example:
What does ARM stand for in an ARM CPU?
Be
sure to watch all 4 videos before class on Monday.
Video
- Intro to Computing Platforms (you might want to watch this
twice, maybe take notes)
How
a CPU Works
(nice graphics)
Operating
System Basics
(overly technical, but informative)
https://www.youtube.com/watch?v=rSyGpxjBGnA
(Nanotechnology for the Future)
~~~~ Questions ~~~~~~~~~
- What does ARM stand for?
Advanced Risc Machines (just ask Google)
- Why/how do ARM processors use less power?
Answer
here (from typing the question into Google)
- Are all microprocessors x86 compatible?
No, they are not. Compatibility means they
accept programs written in the same MACHINE LANGUAGE.
Many CPUs are indeed x86 compatible (e.g. AMD),
but most CPUs are not. Many special purpose CPUs
are made for devices like Smartphones (ARM), GPS, medical equipment.
These must be considerably simpler because they
must consume less power.
- How do numbers get into the RAM?
via the Data Bus.
Try the Little Man CPU Sim for
a clear demonstration.
Here
is an interesting artical about x86 vs ARM architectures.
~~~~~~~~~~~~~~~~~~~~~
ppt
by Liu UCDavis
8080
CPU Video
200
Videos about Microprocessors
Here
is a history of developments in x86 microprocessors.
We will look at some CPU simulators, to get a better concept
about what is going on at the hardware level.
Little
Man CPU Sim
6502 Simulator
Videos About Platforms - 28 Jan 2016
Video - Intro to
Computing Platforms (you might want to watch this twice, maybe take
notes)
How a CPU Works
(nice graphics)
Operating System
Basics (overly technical, but informative)
https://www.youtube.com/watch?v=rSyGpxjBGnA
(Nanotechnology for the Future)
== Homework for Thursday Evening and Friday
Evening =================
Watch the FIRST HALF of each of the 4 videos above.
Sit with a pen and paper and WRITE DOWN questions
that arise during viewing. For example:
What does ARM stand for in an ARM CPU?
Be sure to watch all 4 videos before class
on Monday.
========================= videos for later
========================================
History of Computing
Hardware
ppt
by Liu UCDavis fetch-execute
animation Little
Man CPU Sim
10
CPU Myths
Computer Systems - Hardware and OS
Systems
and Hardware Pictures
Discussing Platforms (notes below) - 26 Jan 2016

Solutions to Programming Practice and Multi-platform Apps
- 25 Jan 2016
Here are solutions to the Programmming Practice
we did last Wednesday.
ProgrammingPracticeSolutions
=== Quiz Today - 20 min ===
== Reference Articles (optional, read as
needed)
Cross-Platform Apps
Java for Cross Platform Mobile
https://adtmag.com/blogs/dev-watch/2015/02/juniversal-project.aspx
Here is an explanation of how to enable Java Applets in various browsers:
https://java.com/en/download/help/enable_browser.xml
Here is a brief explanation of "cross platform" software.
https://www.techopedia.com/definition/17056/cross-platform
Notes about porting Java from Mac to other platforms
http://www.mactech.com/articles/mactech/Vol.14/14.05/WritingJavaCross-Platform/index.html
Java Issues
http://www.makeuseof.com/tag/top-6-install-java-software/
Naughty Twins - Java and Flash
https://www.linkedin.com/pulse/its-naughty-step-again-terrible-twins-java-flash-william-buchanan
Java Security Issues
http://www.infoworld.com/article/2613631/security/java-s-security-problems-unlikely-to-be-resolved-soon--researchers-say.html
http://www.computerworld.com/article/2474497/mac-os-x/apple-closes-java-hack--and-why-it-s-time-to-switch-java-off.html
http://www.wiley.com/legacy/compbooks/press/mcgch1.html
Google Inbox for Mobile Apps
http://arstechnica.com/information-technology/2014/11/how-google-inbox-shares-70-of-its-code-across-android-ios-and-the-web/
Why Mobile Apps are Slow
http://sealedabstract.com/rants/why-mobile-web-apps-are-slow/
Chrome
Running Android Apps on other Platforms
http://www.omgchrome.com/run-android-apps-on-windows-mac-linux-archon/
Switch Now - it's Easy
http://www.makeuseof.com/tag/make-an-easy-switch-to-chromebook-now-and-never-look-back/
Switch to a Tablet - you don't need a PC
http://www.howtoreplaceyourpc.com/the-tablet-all-in-one/
CPU Compatibility
http://www.howtogeek.com/180225/arm-vs.-intel-what-it-means-for-windows-chromebook-and-android-software-compatibility/
(ARM vs Atom)
https://kb.wisc.edu/page.php?id=4927
(Intel, AMD, Arm)
http://www.tomshardware.com/t/cpus/
(Too Much Info about CPUs)
Attendance Program - 22 Jan 2016
Today we will use LIST controls to create a simple ATTENDANCE program.
*** QUIZ on Monday 25 Jan ***
The quiz will be similar to the last one -
you will use your computer to write a short
program and then copy it onto paper.
The programming problem will be
similar to the problems you programmed on Wednesday.
[HL] Operating Systems - 21 Jan 2016
We will briefly discuss the Extended Essay, especially how it relates to
Computer Science.
Then we will use Hypnosis to study how MULTI-TASKING and MULTI-THREADING
work.
== Homework ==
Read
about Operating Systems
Optional : watch
this video
Programming Practice - 20 Jan 2016
Practice programming today.
Read
these notes and do the suggested exercises.
More Discussion of OOP and Gregorian Calendar - 19 Jan
2016
public, private, protected
static, final,
etc
Java
Keywords with Examples (reference, use whenever it is helpful)
Gregorian Calendar - 18 Jan 2016
(1) Quiz
about Dates
(2) Discuss the GregorianCalendar class, using BlueJGUI7.zip
Here is a reference tutorial with lots
of details and examples:
https://www3.ntu.edu.sg/home/ehchua/programming/java/DateTimeCalendar.html
(3) == Homework ==
Read p.120-124 in Wu
Java Book
More Time and Date Problems - 14 Jan 2016
(1) Make a new program that contains a STOP WATCH.
It needs a [Start] button and a [Stop] button.
When the [Start] button is clicked, the program
makes a new Date() variable to remember the
Starting time.
When [Stop] is clicked, it makes a new Date() to
remember
when it was clicked. Then it
outputs the total amount
of time between Starting and
Stopping. It must use .getTime()
to get milliseconds for each of the
times, and then
subract them, and then convert the
result to FRACTIONS of SECONDS.
So the result might be 1.72 seconds.
(2) Find many different LEGAL ways of typing (inputting) a Date.
Here are a few: 12 Jan 2016 ,
1/12/16 , 12.1.2016
Make a list of formats that WORK and others that
DON'T WORK.
(3) Input someone's birthdate (day, month and year) and then
output the Day of Week that they were born (e.g.
Sunday for 23 Aug 1953).
(4) Input two times (like 8:32 and 15:10) and calculate the number
of MINUTES between the two times. Do this
with new Date() variables.
*** 20 min Quiz on Monday 18
Jan ***
*** You may use your computer and ***
*** all your notes during the quiz. ***
Here is a solution for the StopWatch program:
/**
* A
simple stopwatch, with [Start] and [Stop] buttons
*
*
@author Dave Mulkey
*
@version 15 Jan 2016
*/
import
java.awt.*;
import
java.awt.event.*;
import
javax.swing.*;
import
java.util.*;
import
java.text.*;
// these 5
PACKAGES contain most of the GUI stuff
public
class StopWatch extends GUI
{
public static void main(String[] args)
{
new StopWatch(); // makes an actual OBJECT
}
// addButtons and other COMPONENTS here
Button start = addButton("Start",50,50,100,50,this);
Button stop = addButton("Stop",150,50,100,50,this);
TextField amount = addTextField("",50,100,200,50,this);
// GLOBAL VARIABLES go here
long started, stopped, elapsed;
public StopWatch() // constructor - this runs
first
{
super(400,300); // initialize GUI and set window
size
// change Fonts and other appearances here
amount.setFont(new Font("Arial",0,24));
// initialize any SETTINGS or VARIABLES here
}
public void actions(Object source,String command)
{
// respond to EVENTS (buttons) here
if(source == start)
{
Date now = new Date();
started = now.getTime();
amount.setText("started");
}
else if(source == stop)
{
Date now = new Date();
stopped = now.getTime();
elapsed = stopped - started;
double seconds = elapsed / 1000.0;
amount.setText(seconds + "");
}
}
}
|
OOP with BlueJ - using Dates - 12-13 Jan 2016
You will need a copy of this BlueJ project : BlueJGUI7.zip
We will be discussing the use of the Date class from the java.util.*
package.
Homework Reading:
Wu
Java Book section 2.4.3
[HL] Bye-bye Kings, Bye-bye Processing - 18 Dec 2015
Here is the final, unbeatable (?) Kings
program
It's very good, but not perfect.
We need to finish it.
New IDE = BlueJ - 17 Dec 2015
**** HOMEWORK *****
Make sure you have BlueJ installed and working
before returning to class on 12 January 2016.
We will now start writing STANDARD Java programs,
without the help of Processing. We will use BlueJ,
an IDE especially designed for students to learn OOP.
Read
these introductory notes about BlueJ.
Getting Started
To get started, you should download 2 files:
- BlueJ IDE at http://bluej.org
Get the version WITH Java 8 SDK
- BluejGUI
example program
The teacher will demonstrate how to use BlueJ,
and explain how the BluejGUI example program works.
Here are some
sample projects.
Here is a video with a
basic introduction for writing your first program
Here is a printed introduction, just like the video
Here is a brief
user's manual, in case you want to learn more about BlueJ
Here is a textbook about BlueJ and OOP
Objects
First with Java: A Practical Introduction Using BlueJ
Continue Memory Game Project - 9-16 Dec 2015
Here
is a MEMORY game - at least a START.
This is a start of a MATCHING game.
Click a square to "show" it's contents.
Then click another square, hoping to get a match.
This is only a start of a game. It needs lots of improvements.
- It uses random numbers. There is no guarantee that
there are exactly 2 of each number. So after a few pairs
are found, no more are possible. It needs to start with
2 ones, 2 twos, 2 threes... 2 eights.
- There should be 2 players who are taking turns.
Whenever a player turns over a pair, they should win points.
At the end, the player with the most points is the winner.
- It would be more challenging with any of the following:
- bigger numbers, like 10..99 instead of 1..9
- the goal is to find pairs that ADD UP to some specific amount,
so it would contain 8 and 7, 6 and 9 , 10 and 5 , etc.
so pairs that add up to 15.
- the players can change the size of the board,
the size of numbers, or the rules each time they
play -
but don't permit an ODD number of size of the board
- if you wish to allow ODD board size, then disable
the middle square
- it should not be possible to click numbers that have
already been matched or to score points that way
- allow several players, not just 2 players
- you can use words instead of numbers. To do this:
- make an array containing words
- when the program chooses a random numbers,
it puts the corresponding WORD into the
button
instead of putting the number in the button
- add a cheat/joker button that reveals a matching number
when it is clicked - but can only be used once per
game
Use more interesting colors to make the game more attractive.
== MEMORY GAME ASSIGNMENT ==
Your assignment is to make a MEMORY game.
You may start with the example above.
Then make improvements as described -
or make other improvements, as you wish.
Your finished project must function correctly
according to your design and your rules.
== DUE DATE = Wed 16 Dec at 18:00 ==
To turn in your program, you must:
- add COMMENTS (in the program listing) that explain
- the rules/how it works
- anything that does not work reliably
- anything the user needs to know to play
- COMPRESS the folder
- upload the .zip archive into Haiku in the MatchingGame assignment
[HL] More Kings - 8 Dec 2015
Here is ver
2 of the Kings program
This version prevents illegal moves.
And here
is a GUI version.
** HOMEWORK **
Try to complete the following before next HL class (Fri 18 Dec)
// Change the GUI program so that:
// the computer plays first
// the computer ALWAYS plays
its FIRST move in the MIDDLE
// Then, after the person
moves, the computer makes
// it's next move
as the REFLECTION through the middle
// It's enough to make it work on a 5x5
board,
// but if you do bigger
boards, even better
// You should probably stick
to ODD sizes
// 6x6 is significantly more
complex
OOP TicTacToe with Larger Boards - 7 Dec 2015
Here is a program
for 4x4 TicTacToe if you are interested.
Here
is a MEMORY game - at least a START.
== MEMORY GAME ASSIGNMENT ==
Your assignment is to make a MEMORY game.
You may start with the example above.
Then make improvements as described -
or make other improvements, as you wish.
Your finished project must function correctly
according to your design and your rules.
== DUE DATE = Wed 16 Dec at 18:00 ==
To turn in your program, you must:
- add COMMENTS (in the program listing) that explain
- the rules/how it works
- anything that does not work reliably
- anything the user needs to know to play
- COMPRESS the folder
- upload the .zip archive into Haiku in the MatchingGame assignment
OOP TicTacToe - 4 Dec 2015
*** 10 Min Quiz ***
Then we will look at an OOP
version of TicTacToe.
== HOMEWORK ==
Spend about 30 min at home trying to make a 4x4 TicTacToe game.
You probably won't finish, but then you will be prepared
for the discussion on Monday during class.
Students Leaving List - 3 Dec 2015
Here is a completed
version the Hotel Program as the teacher wrote during class.
Today we will work on this program: Students
Leaving List
Hotel Reservations - 1 Dec 2015
This program records reservations for a small hotel, with only 9 rooms.
We will work on this for several days and use various OOP techniques.
Download
Hotel Program
OOP Continued - 30 Nov 2015
Continue with Composite
Data Structures and OOPparking.zip
== HOMEWORK ==
Read sections 1.1 and 1.2 and 1.3 in Wu
Java Book
Starting Object Oriented Programming - 25 Nov 2015
We will discuss these notes: Composite
Data Structures
and study this sample program : OOPparking.zip
KINGS - a 2D Board Game - 24 Nov 2015
How do board games work?
How can we store Tic-Tac-Toe or Mine Sweeper or KINGS in Java?
Here is a game (simulation) to explore: KINGS
HL Homework -
Before next HL meeting, become a KINGS expert -
analyze the game and develop a winning STRATEGY.
Arrays Test + Starting Objects - 23 Nov 2015
*** Test about Arrays - will last 30-50 min (depending on your speed) ***
== HOMEWORK ==
Read : Composite
Data Structures
We will work on the Practice Program on Wednesday
TicTacToe using a Simple Array - 20 Nov 2015
TicTacToe
Game - do the practice exercises at the end of the program
*** Test on Monday - written on paper, no
computers - 50 min ***
Searching in Parallel Arrays - 19 Nov 2015
Solutions
for yesterday's CALENDAR program (also needs the IO methods)
More practice with PARALLEL
ARRAYS
More Array Programming Practice - 17 Nov 2015
Solutions
for yesterday's assignment
Assignment for today:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/calendar.zip
Programming Practice - 16 Nov 2015
We need to do some more programming practice this week.
We will start with this assignment:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/ArrayMethods/ArrayMethods.pde
*** TEST ANNOUNCEMENT ***
We will have a TEST (50 min) next Monday, 23 Nov 2015.
It will be all about programming algorithms involving arrays of numbers,
including sorting, searching and changing numbers in arrays.
Help With Packing Boxes - 13 Nov 2015
Here
is the latest Packing Boxes Program.
Now we will work on a HELP
algorithm that gives helpful information, like:
- how much space is left in the current box?
- what is the largest number in the list that will fit into the current box?
- are there 2 numbers in the list that will exactly fill the current box?
- are there 3 numbers in the list that will exactly fill the current box?
Also useful would be an algorithm that SORTS
all the numbers into descending order.
[HL] Elevator Logic - 12 Nov 2015
- Here is the WORST WEB-SITE
EVER with a usable
Simulator.jar.
- Here is a video
of an Elevator Control Simulator.
- Here
is the teacher's attempt at a smarter elevator program.
- download the program
- run it several times. Find out what works and what doesn't
- add more commands to make more stuff work correctly
- Here
is an essay (too long for Extended Essay) about Elevator Control.
- Here
is a Design Document for an elevator control program (sort of like
an IB IA Project)
Packing MORE Boxes - 11 Nov 2015
Continue with this program : Packing
Boxes problem
Here is an OPTIONAL reading assignment,
explaining the Knapsack Problem and how
it can be used for Public Key Encryption.
http://nrich.maths.org/2199
*** [HL] HOMEWORK **
The [HL] homework from last class
was to improve this elevator program:
Simple
Starting Program
[HL] class meets tomorrow.
Bring your improved program with you.
Packing Boxes - 10 Nov 2015
Try this program for the Packing
Boxes problem.
Solving Puzzles with Num Lists - 9 Nov 2015
Consider the following problem:
The following weights must be shipped : 160
140 120 110 90
90 60
They can be shipped in 2 cars, each of which can carry up
to 300 maximumum.
How should the weights be distributed for shipping?
*** Homework ***
Estimate HOW MANY DIFFERENT SOLUTIONS for this problem are possible,
including VERY BAD solutions like:
- 60 in one car, 90 in the other, and don't ship 160,140,120,110,90
as well as good solutions like:
- 160 + 140 in one car, 120 + 90 + 90 in the other car, don't
ship 110,60
as well as the ALMOST good solution:
- 160 + 140 in one car, 120 + 110 + 60 in the second car, don't ship
90,90
Tracing Practice - 5 Nov 2015
Tracing
Practice
Tracing
Practice Solutions
** 20 min Quiz on Monday - Tracing Array
Algorithms **
More Sorting Algorithms - 4 Nov 2015
Sorting
and Other Algorithms for an Array of ints
Sample
Sorting Program
Java Code with Visual Trace
***HOMEWORK***
Make the BubbleSort method work in the Sample
Sorting Program

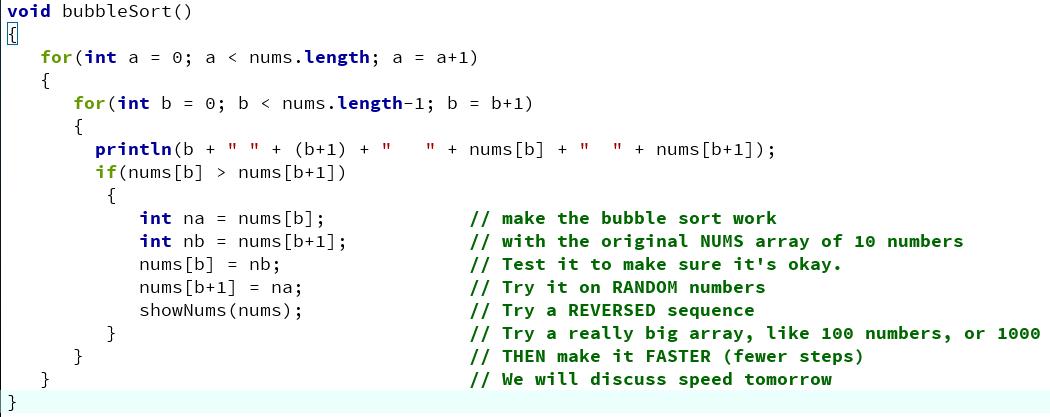
Sorting Algorithms - 3 Nov 2015
Here
are solutions to the Number Arrays Practice program.
The previous program had difficulty finding the Median of an array of
numbers.
This should be easy, as the median is simply the middle number in a sorted
list.
However, sorting the list is not so simple. We will study several
algorithms for sorting an array (putting it in order).
Visual
Sorting Algorithms Demonstration
Java Code with Visual Trace
Sorting
and Other Algorithms for an Array of ints
Sample
Sorting Program
[HL] Elevator Diagrams - 2 Nov 2015
Sample CASE
STUDY with several diagrams.
How can we build a SIMULATION program?
Finite State
Machines
ATM Intro to State
Diagrams
Here is a simple State Diagram for an elevator:

Simple
Starting Program
*** HOMEWORK ***
Before next [HL] class, you need to
- download the program
- add the changes that the teacher did in class
- add as much as you can to make the simulation better,
working at least 1 hour before next class
- bring QUESTIONS and PROBLEMS to the next [HL] class
Number Arrays Practice - 29 Oct 2015
Download
this program.
Make the changes described in the code,
as well as any other changes that seem sensible.
Do NOT replace these algorithms with your own version -
you must DEBUG (fix) the code that already exists.
More Numbers in Arrays - 27-28 Oct 2015
We need to write algorithms for all the following for an ARRAY full of
NUMBERS:
- count the number of data items larger than 10
- search for a specific number and count how many times it occurs
- search for two numbers that add up to exactly 100
- search for DUPLICATES
- find the TWO LARGEST numbers
- find the TOP 10 NUMBERS
- print a FREQUENCY DISTRIBUTION in specifc classes
... more tomorrow ...
We wrote this in class:
double[]
ages = { 15.9999999999999,16,17,16,17,3,12,15,16,17,16 };
void setup()
{
int answer =
countOver(16); // 10 is a PARAMETER
println(answer);
}
int countOver(int
min) //
camelCaps
{
int count
= 0;
for(int
c=0; c < ages.length; c++)
{
if( ages[c] >= min
)
// choice
{
count = count + 1;
}
}
return
count;
}
|
Arrays for Numbers Data - 23 Oct 2015
We will write a program that does Statistical calculations with a list of
numbers.
Download
the starting program here.
** HOMEWORK **
Download the program and do the practice exercises at the end.
Spend about 30 minutes and finish as much as you can.
We will continue on Tuesday.
== Extra Programming Practice ==
If you started the IB Computer Science course with no previous
programming experience, you probably need extra practice.
There are many books and tutorials available for learning Java.
They are generally not written for High School students,
and certainly not designed for IB Courses.
This
set of practice programs
was written specifically for IB students.
You can do one lesson at a time, without doing other lessons.
Quiz about WordList Program - 22 Oct 2015
Searching Practice - 21 Oct 2015
Download
this program and do the practice exercises at the end.
You will also need the [IO] library.
Download
this .zip archive if you prefer.
[HL] Elevator Control Logic - 20 Oct 2015
We will discuss the LOGIC DESIGN that students did for homework.
Airports List - 19 Oct 2015
Today start with this improved program:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/wordlist2.zip
and use this data : Airports.txt
We will continue tomorrow, and have a 20 min quiz on Thursday about
Searching String Arrays.
Here is most of the code that Mr Mulkey typed to do better searches,
including 2 words.
** HOMEWORK ** Type
this into the WordList program and make it work correctly.
boolean found =
false;
String target =
input("Type word(s)");
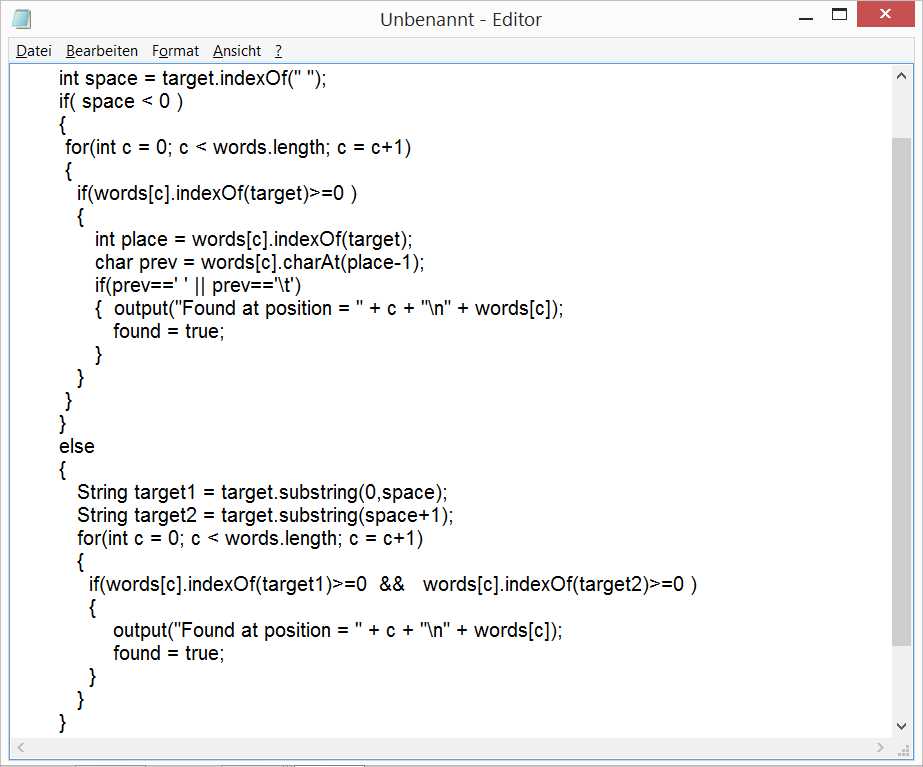
if( found == false )
{
output("Not found");
}
Continue Word Lists - 16 Oct 2015
*** Homework ***
- Quiz Monday - you will need to write a correct SEARCH ALGORITHM
just like the one in the wordlist2 program below - maybe
memorize it
- Think about how we can make a search method
that can search for a city in the Airports list (below)
***************
Today start with this improved program:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/wordlist2.zip
We will concentrate on SEARCHING, using the following data files:
Randomly sorted words : this
random list of words.
Here is a list of names by popularity: Given
Names.txt
Here is a text file for future use: Airports.txt
Word Lists - 15 Oct 2015
We will be using this
list of words
and this
program.
We will be learning about:
- arrays
- sequential search
- binary search
Here is an improved version of the program including a search box:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/wordlist2.zip
We might also use this
random list of words.
Here is a list of names by popularity: Given
Names.txt
Here is a text file for future use: Airports.txt
Secret Code Program - 13 Oct 2015
Box start,crypt;
String plainText = "secret message for today"; // input("Type the
plain text");
void setup()
{ size(600,600);
IOstart();
start = new Box("Encrypt",20,20);
crypt = new Box("",20,60,300,30);
}
void draw()
{
showBoxes();
}
void mouseClicked()
{
if(start.isClicked())
{
crypt.setText(
encrypt(plainText) );
}
}
String encrypt(String plain)
{
// split up the letters .substring
// change ASCII codes by adding 1 or 2
String result = "";
for(int c = 0; c < plain.length(); c = c + 1)
{
char ch = plain.charAt(c);
char nch = (char)(ch + 1);
result = result + nch;
}
return result;
}
/********************************
Basic TEMPLATE = every program needs this
*********************************/
Math Sequences - 12 Oct 2015
Here is a solution to the problems from last class:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/EasyDice4.zip
Today we will study a program that prints mathematical sequences.
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/EasySequences.zip
MOOOOOOOOOOORRRRRRRE Dice - 9 Oct 2015
Download
this program and do the exercises listed at the end.
[HL] Elevator Program - 8 Oct 2015
ElevatorControl
Program
We will listen to some STORIES
about how elevators work,
and then continue our program development.
== Homework ==
Design LOGIC for a 20 floor building, 1 single elevator.
Present your LOGIC in any fashion you find sensible.
Focus on the question "WHAT IF??"
But please don't write a Java program (unless you find that easy).
Due next HL meeting.
Your solution should deal sensibly with
the USER STORIES we heard:
USE CASES == Stories == WHAT IF
Person A wanted to get to level 6 but
had to wait for the elevator to go down
to level 1, making him unhappy.
Workung on 10th floor, needs to go
down to 1, the elevator stops several times
maybe that could be random every time.
Person gets in at 5, going to 2nd floor,
and then people get in at 3 and want
to go up to 4.
Bank robber running from police.
Gets into elevator and goes to 12,
but changes at 6, goes to 8,
the police use the same elevator
and go to 12, but security have
him already but cannot use the elevator.
A child enters the elevator, presses
all 10 buttons for fun.
5 person weight limit, 5 people in
elevator, someone falls onto the top
from the 6th floor through shaft.
There is a thunderstorm and power
goes off and elevator gets stuck ****
Empty elevator arrives at 3 in 6 story
building with both up and down pushed outside
and it must decide which way to go.
There are brakes to control the speed. |
GUI with Pictures - 7 Oct 2015
Download this program: EasyDice2.zip
Run the program. Then make the improvements stated at the end of the
program.
Using GUI Interface - 6 Oct 2015
Download this program: EasyDice1.zip
Run the program. Then make the improvements stated at the end of the
program.
String Practice - 23 Sep 2015
Here is a solution to yesteday's fraction program:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/Fractions1answers/Fractions1answers.pde
Practice for your Strings test (Friday) be doing the following problems:
Problems : https://dl.dropboxusercontent.com/u/275979/ibcomp/Strings/StringPractice.html
Answers : Here
are answers for these practice problems.
Fractions - 22 Sep
2015
Here is an answer to Swastik's question about updating
money exchange rates on a daily basis:
http://www.xe.com/xecurrencydata/
We will do some programming practice with FRACTIONS:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/Fractions1/Fractions1.pde
Parsing Strings - 21
Sep 2015
Changing
Money
Elapsed
Time
We will discuss PARSING (chopping up) Strings to make
input easier.
Savitch Section 1.3
[HL] Elevator Logic -
18 Sep 2015
We are going to (quickly) write an Elevator Simulator,
where we can experiment with the LOGIC of elevator control.
ElevatorControl
Program
== HOMEWORK ==
Before the next [HL] class, write STORIES
about an elevator.
Spend at least 30 minutes and describe
SITUATIONS
that affect the behavior of the elevator.
Whenever you are using an elevator, THINK
ABOUT
what the elevator is doing and what decisions
it must make -
this will help you write stories.
String Methods - 15-17
Sep 2015
String
Notes and Practice
String
Methods and Functions
More Strings and
String Functions - 11 Sep 2015
Commands
Program
** Homework **
Download the Commands program and add a few
morhttps://dl.dropboxusercontent.com/u/239179/ibcomp/Strings/StringExamples.htmle commands, like:
- subtract
- multiply
- factorial
- tell a joke (using output)
Strings - 10 Sep 2015
== Homework ==
- find the UNICODE value for this
symbol: ☒
- ASCII
- find out what it means
- state one specific example, like the ASCII Code for A is 65
- find out why it was (and still is) important
- how many BITS do ASCII symbols use?
- UNICODE
- find out what it means
- explain why it is important
- Remember your answers for TOMORROW'S
short quiz
- Add some more English/German
translations to the sample program below
and make sure it works correctly in Processing.
Source
code here or BIG
source here
void setup()
{
size(200,200);
String word =
input("Type an English word");
if(
word.equals("food") )
{
output("Essen"); }
if(
word.equals("happy") )
{
output("glücklich"); }
if(
word.equals("money") )
{
output("Geld"); }
}
public String input(String prompt)
{ return
javax.swing.JOptionPane.showInputDialog(null,prompt); }
public void output(String message)
{
javax.swing.JOptionPane.showMessageDialog(null,message);
}
|
Here are two more programs with String variables:
Converting
Units
Password
Check
[HL] Embedded
Processor Devices - 8 Sep 2015
== Presentations ==
Heating system - Gabi Sean
Taxi meters - Tommy Rodion
Elevators - Etienne Lewis
Domestic Robot - Angelo Julian
GPS - Barak Jack
Traffic lights - Swastik Kyle
Smart watches - Andrew Haruki
Drones - Harsh Julius
Washing machine - Mulkey
== Washing Machines ==
Sensors
and Actuators in Washing Machines
More
notes about Washing Machines
Do
It Yourself Project for SMS Alert
Computational Thinking
and Mathematical Models - 7 Sep 2015
Solve
Math Equation - falling rock MODEL
Ski
Trip Model
Converting
Units of Measure (we will discuss this in our
next class)
Doubles, Floats and
Ints - 4 Sep 2015
Finish Calculations
+ - * /
Discuss Bake
Sale
Practice Solve
Math Equation
Start Java Programming
- 3 Sep 2015
**HL Reminder
*************************************************
The next HL class is on Tuesday. You
and your partner
should be prepared to explain your device
(see list of devices and students below)
********************************************************************
Today we will start writing Java programs, with
calculations.
You need to download and install Processing from http://processing.org
(or borrow a USB stick from the teacher). You
should use the
latest version - 3 Beta (#5).
We will start with some calculations:
https://dl.dropboxusercontent.com/u/275979/ibcomp/processing/Calculations/index.html
These have similar problems to Pseudocode,
plus some more problems related to INTEGER number (int),
because they are stored in BINARY. These teacher will
explain further details - you should take notes.
You should complete the practice exercises in the link
above.
You may find Chapter 3 in the following reference book
helpful:
Wu
OOP Java
Binary Practice - 28
Aug 2015
Here is a review of binary intro : Counting
in Binary (video)
Here are some interesting Binary
Storage Examples
Here are some practice
calculations in Binary
[HL] Control Systems
in Common Devices - 27 Aug 2015
== HOMEWORK ==
Before next HL meeting (next G day)
find out HOW YOUR DEVICE WORKS
by searching in Google and reading about it.
Be prepared to explain your information to the class.
Answer at least #1-#4 below (maybe more).
Heating system - Gabi Sean
Taxi meters - Tommy Rodion
Elevators - Etienne Lewis
Washing machine - Mulkey
Domestic Robot - Angelo Julian
GPS - Barak Jack
Traffic lights - Swastik Kyle
Smart watches - Andrew Haruki
Drones - Harsh Julius
- What input devices (sensors)
must exist in the system?
- What output devices (actuators/transducers
or motors) must exist?
- What processing must occur to make the system
function correctly (input-processing-output)?
- What rules must be implemented as decisions in the
embedded controller?
IB
Computer Science Course Guide p.39
7.1.1 Discuss a range of control
systems.
A variety of control systems should be examined such as:
- automatic doors
- heating systems
- taxi meters
- elevators
- washing machines
- process control
- device drivers
- domestic robots
- GPS systems
- traffic lights
. . . and other common devices.
Technical knowledge of specific systems is not expected
but students should be able to analyse a specified
system.
7.1.2 Outline uses of microprocessors
and sensor input
in control systems.
~~~~~~~~
We will study some sample control systems.
In each case, consider the following questions:
- What input devices
(sensors) must exist
in the system?
- What output devices
(actuators/transducers or motors) must exist?
- What processing must occur to make the system function
correctly (input-processing-output)?
- What rules must
be implemented as decisions in
the embedded controller?
You might want to think about processing
first,
and then think about inputs and
outputs.
For the most part, there is little storage in embedded controllers,
or at least nothing interesting.
More Numbers and
Calculations - 26 Aug 2015
What causes the errors in the Pseudocode Calculation
program?
They are caused by the use of BINARY to store numbers in computers.
The teacher will explain this. Here are some extra
notes if you need to read more:
Computer
Science Illuminated - Chapter 2
Binary
Conversions
Numerical
Error Disasters
Computation = Abstraction
+ Automation - 25 Aug 2015
We will start discussing PROGRAMMING as a method to
use Abstraction and Automation to implement Computation Thinking.
We will be using this Pseudocode
Tool .
HOMEWORK:
- Find the largest PRIME NUMBER
that is still smaller than 1 million
- Find the largest number that can
be typed into a Pseudocode command like this:
output 1e100
That one works, but the following does not
output 1e1000000 → Infinity
More about Textbooks - 24
Aug 2015
Today's class:
1 - Finish collecting bar-codes for textbook
registration
2 - Discuss how to use the Illuminated
textbook
3 - Some more reading here:
Understanding
Computers Today and Tomorrow
Discussing System
Scenarios - 20 Aug 2015
Today's class:
1 - Distribute Textbooks
2 - Discuss the "Textbook System"
3 - Discuss the scenarios in Computer
Systems Notes
First Day - 19 Aug 2015
Today's Assignment
How do we define the
term COMPUTER?
We will discuss these notes Computer
Systems Notes .
** Homework **
- Read chapter
1.1 in your textbook.
==== Read the information below as time
permits ====
Partition Problem
https://www.youtube.com/watch?v=9k9xL9w7-3w
Welcome to IB
Computer Science
In IB Comp Sci we
will be writing programs in Javascript, as well as learning
technical concepts and vocabulary about computer
systems
..
more ..
|

|
Daily Work - Computers Required - Bring Your Laptop to Class Every Day
Each student needs a computer for class every day ..
more ..