Table of Values
Download Source Code
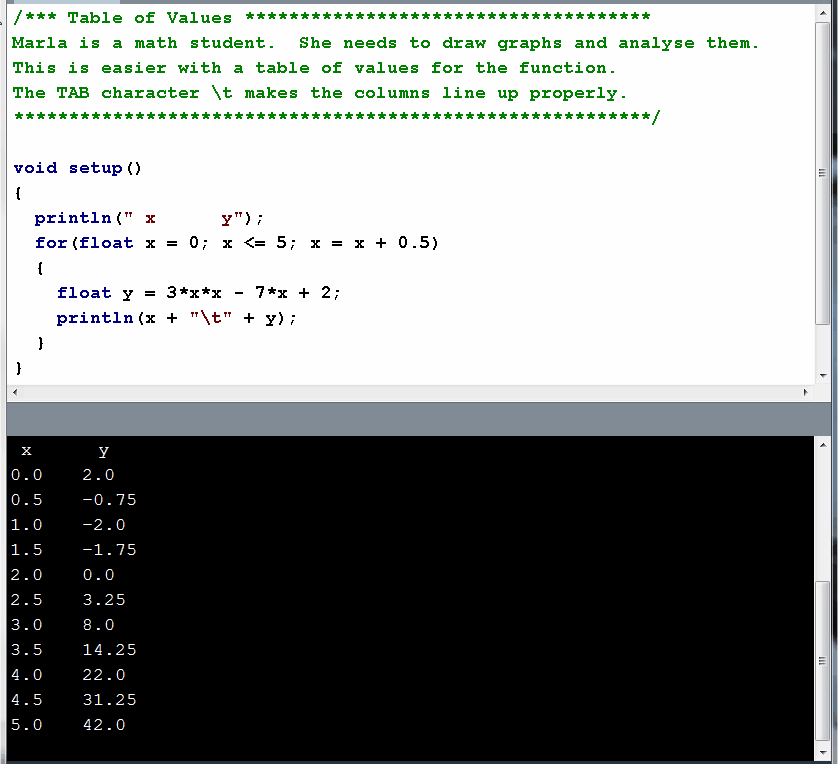
Loops
A loop causes commands
to repeat over and over again.
for(float x = 0; x <= 5; x = x + 0.5)
The for(...) loop causes
the variable X to :
start counting at X = 0
keep counting while (as long as) X is smaller or equal to 5
add 0.5 before repeating
So X has the following values: 0 , 0.5 , 1.0 , 1.5 , 2.0 ,
2.5 , ..... , 4.5 , 5.0.
At each value of X, the value of Y is calculated and then X and Y
are printed.
The control character "\t" is use to create a TAB that lines up
the values in two columns.
This creates a table of values
as shown in the output box above.
Practice
- Download the program, run it and check that the output is
correct.
- Change the Y calculation to calculate y = 3x - 4
- Change the for...
command so that X counts : 0 , 1 , 2 , 3 , .... 10
- Change the program to produce this table of values:
x y
= x*x - x
5
20
6 30
7 42
8 56
9 72
10
90
- Change the program so that it asks the user to input the
starting value for X and the ending value for X.
Use the following commands to do the input:
int start =
int(input("Starting value for x"));
int end = int(input("Ending value for x"));
Then change the for.. loop
so that X counts from start
to end.
- Make X count backward, from 10 down to 0, like this:
for(int x = 10; x >= 0; x = x-1)