Math Tools Demo
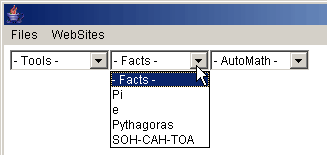
This program demonstrates some ideas that make your program more useable and interesting without any great effort.
The following ideas are included:
(1) Using MENUS to organize your program
(2) Using Choice boxes to organize user commands
(3) using runProgram to execute other programs, making them part of
a system
(4) printing results into a text-file, making them saveable
(5) printing results into an HTML file and using HTML formatting tags
(6) Using HTML files to display user help/instructions
Try changing each of the features, specifically:
(a) Add an ABOUT function
to the FILES menu that pops up a Dialog about the program
(b) Add another
web-site to the Web-Sites menu, and make it appear when clicked
(c) Add a
word-processor to the tools - perhaps WordPad
(d) Add a new MENU containing
2 GAMES - minesweeper and solitaire
(e) Add a new CHOICE box containing at
least 2 commands
(f) Add a method that prints the following sequence into
a text-file: 1,3,5,7,...99
(g) Add a method to make a FORM LETTER with the
user's name, printed to an HTML file.
It should input
several words that get inserted into the text, using some
formatting
tags - something like this:
Complain Letter
To: Mr Gates
From: Your Name Your
address Your
City Germany
Dear Mr Gates
I have purchase MS Office 2003. I
am having trouble running the spell check. Please
refund the purchase price immediately.
Sincerely, Your
Name
|
Click here to download an archive containing the
program, easy app, and help-file(s).
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.*; // contains Day and other Calendar/clock commands
public class MathTools extends EasyApp
{
public static void main(String[] args)
{
new MathTools();
}
Menu mFiles = addMenu("Files|Help|Quit");
Menu mWebSites = addMenu("WebSites|Pascal's Triangle|Time Zones");
Choice cTools = addChoice("- Tools -|Windows Calc|Notepad",10,50,100,30,this);
Choice cNums = addChoice("- Facts -|Pi|e|Pythagoras|SOH-CAH-TOA",110,50,100,30,this);
Choice cAutoMath = addChoice("- AutoMath -|GP|Bars|Print Evens",210,50,100,30,this);
String homeFolder = System.getProperty("user.dir")+"\\";
// finds the path to the folder where the program started
// use this to find data-files stored in the same folder
public void actions(Object source,String command)
{
// EasyApp provides runProgram for executing other programs
// Use runProgram("explorer ...") to open a web-site in IE
// Include homeFolder for when viewing a data file or help file
if (command.equals("WebSites|Pascal's Triangle"))
{ runProgram("explorer http://nlvm.usu.edu/en/nav/frames_asid_181_g_4_t_1.html");}
else if (command.equals("WebSites|Time Zones"))
{ runProgram("explorer http://www.worldtimezone.com/"); }
else if (command.equals("Files|Help"))
{ runProgram("explorer " + homeFolder + "help.htm"); }
else if (command.equals("Files|Quit"))
{ System.exit(0); }
else if (command.equals("Windows Calc"))
{ runProgram("calc.exe"); }
else if (command.equals("Notepad"))
{ runProgram("notepad.exe"); }
else if (command.equals("Pi"))
{ output("Pi = 22/7"); }
else if (command.equals("e"))
{ output("e = 2.71828..."); }
else if (command.equals("Pythagoras"))
{ output("Pythagoras : a² + b² = c²"); }
else if (command.equals("SOH-CAH-TOA"))
{ runProgram("explorer " + homeFolder + "SOHCAHTOA.htm"); }
else if (command.equals("GP"))
{ gp(); }
else if (command.equals("Bars"))
{ bars(); }
else if (command.equals("Print Evens"))
{ String[] numbers = new String[50];
for (int x = 0; x < 100; x = x+2)
{
numbers[x / 2] = x + "";
}
ArrayPrinter printer = new ArrayPrinter(numbers);
}
}
public void gp()
{
// Save results in a text-file. This could allow the user to
// collect lots of results if the filename where changeable
// Opens file in Notepad so users can add notes and save
// under a different name if they wish
double a = inputDouble("Starting Number:");
double r = inputDouble("Common Ratio:");
try
{
String filename = homeFolder + "gp.txt";
PrintWriter outfile = new PrintWriter(new FileWriter(filename));
outfile.println("GP");
outfile.println("a = " + a );
outfile.println("r = " + r );
for (int n=1; n <= 10; n++)
{
outfile.println(a*Math.pow(r,n-1));
}
outfile.close();
runProgram("notepad " + filename);
}
catch(IOException ex){ }
}
public void bars()
{
// Writes results into an HTML file. This allows use of HTML tags
// for formatting. Remember to start and end with <HTML> and <BODY tags
try
{ String title = input("Title:");
String yourname = input("Your name:");
String filename = homeFolder + "bars.htm";
PrintWriter outfile = new PrintWriter(new FileWriter(filename));
outfile.println("<html><body>");
outfile.println("<H2>" + title + "</H2>");
for (int n=1; n <= 10; n++)
{
String name = input("Word (blank to quit)");
if (name.length()==0) {break;}
int num = inputInt("Number");
outfile.print("<font style='background=navy'>");
for (int x = 0; x<num; x++)
{ outfile.print("[]"); }
outfile.print("</font>");
outfile.println(" " + name + " " + num + "<p>");
}
outfile.println("by " + yourname + " , created " + (new Date()) + "<p>");
outfile.println("</body></html>");
outfile.close();
runProgram("explorer " + filename);
}
catch(IOException ex){ }
}
}